How to Implement Verify using cURL
Prerequisites
- A Movider account. If you don’t have one, please sign up first.
- API key and API secret.
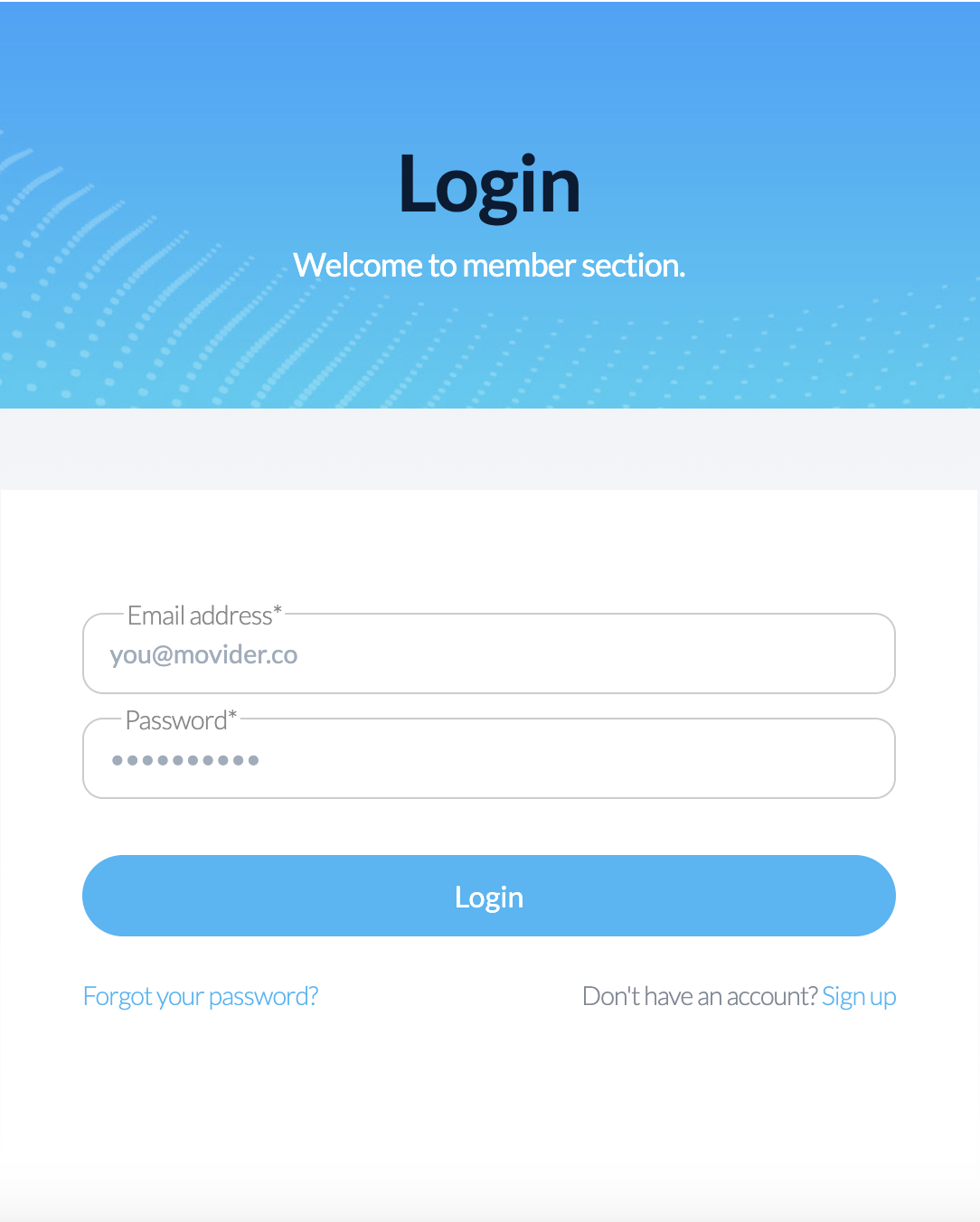
Login to Movider member section.
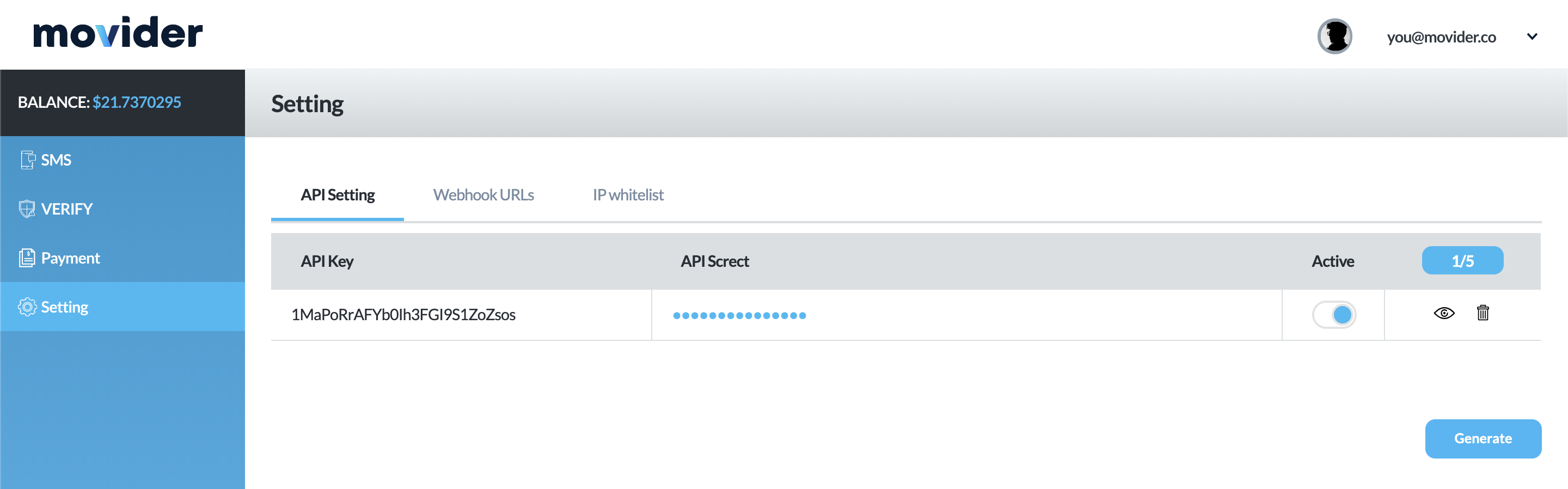
Go to Settings, then you can see your
API key and API secret at API Settings tab.
How Verify Work
First, an SMS message with verification code will be sent to verifying phone number.
Then, the verification code will be used for acknowledge the verification.
If the acknowledged code is matched, the verification succeeded.
If there is no successful acknowledgement within determined period of time, a voice message with verification code will be called to verifying phone number as the second attempt.
If there is still no successful acknowledgement before defined expiry, the verification failed.
Step 1: Sending Verification Code
Take API key and API secret from prerequisite steps, make a request using cURL as shown in below example.
curl -X "POST" https://api.movider.co/v1/verify \
-d to=6678901234 \
-d api_key=1KDFRBMfpbf8184RghTlffnbGT5 \
-d api_secret=tzp99FCPR89o9rMJ••••••••••••••••••••••••
This example request includes required parameters which are to, api_key, and api_secret. More detail explanation and other available optional parameters could be found in API References.
Then you will get the response including the request ID for acknowledge verification in the next step and the destination phone number. See an example of response below.
{
"request_id": "VtznKfqHUjmDCysZyOZDQX",
"number": "66877177214"
}
<xml>
<request_id>VtznKfqHUjmDCysZyOZDQX</request_id>
<number>66877177214</number>
</xml>
The default format of the response is JSON, but you can set it to application/xml for XML using Accept Headers in your request. See more detail in API References.
If you didn’t get the response as expected, please see the Error table in API References for troubleshooting.
Step 2: Acknowledging Verification Code
To acknowledge the verification, take the request_id from Step 1, along with the verification code entered by your user through your application, as well as api_key and api_secret.
curl -X "POST" https://api.movider.co/v1/verify/acknowledge \
-d request_id=VtznKfqHUjmDCysZyOZDQX \
-d code=1234 \
-d api_key=1KDFRBMfpbf8184RghTlffnbGT5 \
-d api_secret=tzp99FCPR89o9rMJ••••••••••••••••••••••••
Response from verification acknowledgement consist of the request ID and the price of successful verification. See an example of response below.
{
"request_id": "VtznKfqHUjmDCysZyOZDQX",
"price": 0.085
}
<xml>
<request_id>VtznKfqHUjmDCysZyOZDQX</request_id>
<price>0.085</price>
</xml>
Cancelling Verification
You can also cancel the verification, please see the example code as following.
curl -X "POST" https://api.movider.co/v1/verify/cancel \
-d request_id=VtznKfqHUjmDCysZyOZDQX \
-d api_key=1KDFRBMfpbf8184RghTlffnbGT5 \
-d api_secret=tzp99FCPR89o9rMJ••••••••••••••••••••••••
The example response could be found below, in JSON or XML.
{
"request_id": "VtznKfqHUjmDCysZyOZDQX"
}
<xml>
<request_id>VtznKfqHUjmDCysZyOZDQX</request_id>
</xml>
For more details about Verify, please see API References.
Updated 3 months ago